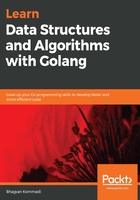
上QQ阅读APP看书,第一时间看更新
The main method – queues
The main method creates two orders, and the priority of the orders is set to 2 and 1. In the following code, the queue will first process the order with the higher number on the priority value:
// main method
func main() {
var queue Queue
queue = make(Queue,0)
var order1 *Order = &Order{}
var priority1 int = 2
var quantity1 int = 20
var product1 string = "Computer"
var customerName1 string = "Greg White"
order1.New(priority1,quantity1,product1, customerName1)
var order2 *Order = &Order{}
var priority2 int = 1
var quantity2 int = 10
var product2 string = "Monitor"
var customerName2 string = "John Smith"
order2.New(priority2,quantity2,product2, customerName2)
queue.Add(order1)
queue.Add(order2)
var i int
for i=0; i< len(queue); i++ {
fmt.Println(queue[i])
}
}
Run the following commands to execute the queue.go file:
go run queue.go
After executing the preceding command, we get the following output:

Let's take a look at Synchronized queue in the next section.