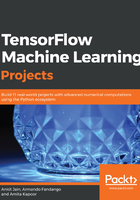
Variables
In the previous sections, we learned how to define tensor objects of different types, such as constants, operations, and placeholders. The values of parameters need to be held in an updatable memory location while building and training models with TensorFlow. Such updatable memory locations for tensors are known as variables in TensorFlow.
To summarize this, TensorFlow variables are tensor objects in that their values can be modified during the execution of the program.
Although tf.Variable seems to be similar to tf.placeholder, they have certain differences. These are listed in the following table:

In TensorFlow, a variable can be created with the API function tf.Variable(). Let's look at an example of using placeholders and variables and create the following model in TensorFlow:
- Define the model parameters w and b as variables with the initial values [.3] and [-0.3]:
w = tf.Variable([.3], tf.float32)
b = tf.Variable([-.3], tf.float32)
- Define the input placeholder x and the output operation node y:
x = tf.placeholder(tf.float32)
y = w * x + b
- Print the variables and placeholders w, v, x, and y:
print("w:",w)
print("x:",x)
print("b:",b)
print("y:",y)
The output depicts the type of nodes as Variable, Placeholder, or operation node, as follows:
w: <tf.Variable 'Variable:0' shape=(1,) dtype=float32_ref>
x: Tensor("Placeholder_2:0", dtype=float32)
b: <tf.Variable 'Variable_1:0' shape=(1,) dtype=float32_ref>
y: Tensor("add:0", dtype=float32)
The preceding output indicates that x is a Placeholder tensor, y is an operation tensor, and that w and b are variables with a shape of (1,) and a data type of float32.
The variables in a TensorFlow session have to be initialized before they can be used. We can either initialize a single variable by running its initializer operation or we can initialize all or a group of variables.
For example, to initialize the w variable, we can use the following code:
tfs.run(w.initializer)
TensorFlow provides a convenient function that can initialize all of the variables:
tfs.run(tf.global_variables_initializer())
The global convenience function for initializing these variables can be executed in an alternative way. Instead of executing inside the run() function of a session object, the run function of the object returned by the initializer function itself can be executed:
tf.global_variables_initializer().run()
After the variables have been initialized, execute the model to get the output for the input values of x = [1,2,3,4]:
print('run(y,{x:[1,2,3,4]}) : ',tfs.run(y,{x:[1,2,3,4]}))
The output is as follows:
run(y,{x:[1,2,3,4]}) : [ 0. 0.30000001 0.60000002 0.90000004]