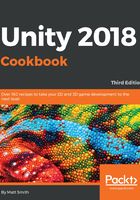
上QQ阅读APP看书,第一时间看更新
How to do it...
To create the simple 2D Space Girl mini-game, follow these steps:
- Create a new, empty 2D project.
- Import the supplied folder Sprites into your project.
- Since it's a 2D project, each sprite image should be of type Sprite (2D and UI). Check this by selecting the sprite in the Project panel, then, in the Inspector, check property Texture Type. If you need to change the type, change it from this drop-down menu, then click the Apply button.
- Set the Unity Player screen size to 800 x 600: choose this resolution from the drop-down menu on the Game panel. If 800 x 600 isn't an offered resolution, the click the plus sign + button and create this as a new resolution for the panel.
- Display the Tags and Layers properties for the current Unity project. Choose menu Edit | Project Settings | Tags and Layers. Alternatively, if you are already editing a GameObject, then you can select the Add Layer... menu from the Layer drop-down menu at the top of the Inspector panel, next to the Static true/false toggle.
- Use the expand/contract triangle tools to contract Tags and Layers, and to expand Sorting Layers. Use the plus sign + button to add two new sorting layers, as shown in the screenshot: first, add one named Background, and next, add one named Foreground. The sequence is important since Unity will draw items in layers
further down this list on top of items earlier in the list. You can rearrange the layer sequence by clicking and dragging the position control: the wide equals sign (=) icon to the left of the word Layer in each row:

- Drag the background_blue sprite from the Project panel (in the Sprites folder) into either the Game or Hierarchy panel to create a GameObject for the current scene. Set the Position of this GameObject to (0,0,0). It should completely cover the Game panel (at resolution 800 x 600).
- Set the Sorting Layer of GameObject background-blue to Background (in the Sprite Renderer component):

- Drag sprite star from the Project panel (in the Sprites folder) into either the Game or Hierarchy panel to create a GameObject for the current scene:
- Create a new tag, Star, and assign this tag to GameObject star (tags are created in the same way we created sorting layers).
- Set the Sorting Layer of GameObject star to Foreground (in the Sprite Renderer component).
- Add to GameObject star a Box Collider 2D (Add Component | Physics 2D | Box Collider 2D) and check Is Trigger, as shown in the screenshot:

- Drag the girl1 sprite from the Project panel (in the Sprites folder) into either the Game or Hierarchy panel to create a GameObject for the player's character in the current scene. Rename this GameObject player-girl1.
- Set the Sorting Layer of GameObject player-girl1 to Foreground.
- Add a Physics | Box Collider 2D component to GameObject player-girl1 .
- Add a Physics 2D | Rigid Body 2D component to GameObject player-girl1 . Set its Gravity Scale to zero (so it isn't falling down the screen due to simulated gravity), as shown in the screenshot:

- Create a new folder for your scripts named _Scripts.
- Create the following C# Script PlayerMove (in the _Scripts folder) and add an instance as a component to GameObject player-girl1 in the Hierarchy:
using UnityEngine; using System.Collections; public class PlayerMove : MonoBehaviour { public float speed = 10; private Rigidbody2D rigidBody2D;
private Vector2 newVelocity;
void Awake(){
rigidBody2D = GetComponent<Rigidbody2D>();
}
void Update() {
float xMove = Input.GetAxis("Horizontal");
float yMove = Input.GetAxis("Vertical");
float xSpeed = xMove * speed;
float ySpeed = yMove * speed;
newVelocity = new Vector2(xSpeed, ySpeed);
}
void FixedUpdate() {
rigidBody2D.velocity = newVelocity;
}
}
- Save the scene (name it Main Scene and save it into a new folder named _Scenes).