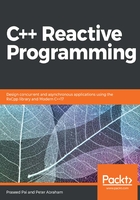
上QQ阅读APP看书,第一时间看更新
Fold expressions
The C++ 17 standard added support for fold expressions to ease the generation of variadic functions. The Compiler does pattern matching and generates the code by inferring the intent of the programmer. The following code snippet demonstrates the idea:
//---------------- Folds.cpp
//--------------- Requires C++ 17 (-std=c++1z )
//--------------- http://en.cppreference.com/w/cpp/language/fold
#include <functional>
#include <iostream>
using namespace std;
template <typename... Ts>
auto AddFoldLeftUn(Ts... args) { return (... + args); }
template <typename... Ts>
auto AddFoldLeftBin(int n,Ts... args){ return (n + ... + args);}
template <typename... Ts>
auto AddFoldRightUn(Ts... args) { return (args + ...); }
template <typename... Ts>
auto AddFoldRightBin(int n,Ts... args) { return (args + ... + n); }
template <typename T,typename... Ts>
auto AddFoldRightBinPoly(T n,Ts... args) { return (args + ... + n); }
template <typename T,typename... Ts>
auto AddFoldLeftBinPoly(T n,Ts... args) { return (n + ... + args); }
int main() {
auto a = AddFoldLeftUn(1,2,3,4);
cout << a << endl;
cout << AddFoldRightBin(a,4,5,6) << endl;
//---------- Folds from Right
//---------- should produce "Hello World C++"
auto b = AddFoldRightBinPoly("C++ "s,"Hello "s,"World "s );
cout << b << endl;
//---------- Folds (Reduce) from Left
//---------- should produce "Hello World C++"
auto c = AddFoldLeftBinPoly("Hello "s,"World "s,"C++ "s );
cout << c << endl;
}
The expected output on the console is as follows
10
25
Hello World C++
Hello World C++