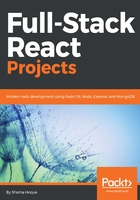
Configuring Express
To use Express, we will first install Express, then add and configure it in the server/express.js file.
From the command line, run the following command to install the express module with the --save flag, so the package.json file is automatically updated:
npm install express --save
Once Express is installed, we can import it into the express.js file, configure as required, and make it available to the rest of the app.
mern-skeleton/server/express.js:
import express from 'express'
const app = express()
/*... configure express ... */
export default app
To handle HTTP requests and serve responses properly, we will use the following modules to configure Express:
- body-parser: Body parsing middleware to handle the complexities of parsing streamable request objects, so we can simplify browser-server communication by exchanging JSON in the request body:
- Install the body-parser module: npm install body-parser --save
- Configure Express: bodyParser.json() and bodyParser.urlencoded({ extended: true })
- cookie-parser: Cookie parsing middleware to parse and set cookies in request objects:
Install the cookie-parser module: npm install cookie-parser --save - compression: Compression middleware that will attempt to compress response bodies for all requests that traverse through the middleware:
Install the compression module: npm install compression --save - helmet: A collection of middleware functions to help secure Express apps by setting various HTTP headers:
Install the helmet module: npm install helmet --save - cors: Middleware to enable CORS (Cross-origin resource sharing):
Install the cors module: npm install cors --save
After the preceding modules are installed, we can update express.js to import these modules and configure the Express app before exporting it for use in the rest of the server code.
The updated mern-skeleton/server/express.js code should be as follows:
import express from 'express'
import bodyParser from 'body-parser'
import cookieParser from 'cookie-parser'
import compress from 'compression'
import cors from 'cors'
import helmet from 'helmet'
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: true }))
app.use(cookieParser())
app.use(compress())
app.use(helmet())
app.use(cors())
export default app