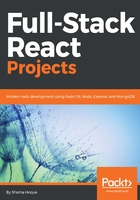
Frontend views with React
In order to start developing a frontend, first create a root template file called template.js in the project folder, which will render the HTML with React components.
mern-simplesetup/template.js:
export default () => {
return `<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>MERN Kickstart</title>
</head>
<body>
<p id="root"></p>
<script type="text/javascript" src="/dist/bundle.js">
</script>
</body>
</html>`
}
When the server receives a request to the root URL, this HTML template will be rendered in the browser, and the p element with ID "root" will contain our React component.
Next, create a client folder where we will add two React files, main.js and HelloWorld.js.
The main.js file simply renders the top-level entry React component in the p element in the HTML document.
mern-simplesetup/client/main.js:
import React from 'react'
import { render } from 'react-dom'
import HelloWorld from './HelloWorld'
render(<HelloWorld/>, document.getElementById('root'))
In this case, the entry React component is the HelloWorld component imported from HelloWorld.js.
HelloWorld.js contains a basic HelloWorld component, which is hot-exported to enable hot reloading with react-hot-loader during development.
mern-simplesetup/client/HelloWorld.js:
import React, { Component } from 'react'
import { hot } from 'react-hot-loader'
class HelloWorld extends Component {
render() {
return (
<p>
<h1>Hello World!</h1>
</p>
)
}
}
export default hot(module)(HelloWorld)
To see the React component rendered in the browser when the server receives a request to the root URL, we need to use the Webpack and Babel setup to compile and bundle this code, and add server-side code that responds to the root route request with the bundled code.