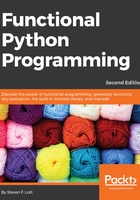
Higher-order functions
We can achieve expressive, succinct programs using higher-order functions. These are functions that accept a function as an argument or return a function as a value. We can use higher-order functions as a way to create composite functions from simpler functions.
Consider the Python max() function. We can provide a function as an argument and modify how the max() function behaves.
Here's some data we might want to process:
>>> year_cheese = [(2000, 29.87), (2001, 30.12), (2002, 30.6), (2003,
30.66),(2004, 31.33), (2005, 32.62), (2006, 32.73), (2007, 33.5),
(2008, 32.84), (2009, 33.02), (2010, 32.92)]
We can apply the max() function, as follows:
>>> max(year_cheese) (2010, 32.92)
The default behavior is to simply compare each tuple in the sequence. This will return the tuple with the largest value on position 0.
Since the max() function is a higher-order function, we can provide another function as an argument. In this case, we'll use lambda as the function; this is used by the max() function, as follows:
>>> max(year_cheese, key=lambda yc: yc[1]) (2007, 33.5)
In this example, the max() function applies the supplied lambda and returns the tuple with the largest value in position 1.
Python provides a rich collection of higher-order functions. We'll see examples of each of Python's higher-order functions in later chapters, primarily in Chapter 5, Higher-Order Functions. We'll also see how we can easily write our own higher-order functions.