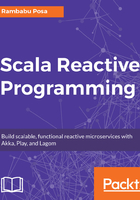
上QQ阅读APP看书,第一时间看更新
A tail-recursion example
Here, we will write a Scala Application to demonstrate the tail-recursion technique.
Scala's @tailrec annotation ensures that tail recursive functions are optimized by the Scala compiler, to avoid StackOverflowErrors:
package com.packt.publishing.recursion object TailRecursionApp extends App { val list = List(1,2,3,4,5,6,7,8,9) val list2 = tailRecursion(list) println("Original List = " + list) println("After Tail Recursion of List = " + list2) @tailrec def tailRecursion[A](listOfElements: List[A]): List[A] = { def reverse(updatedList: List[A], originalList: List[A]):
List[A] = originalList match { case Nil => updatedList case head :: tail => reverse(head :: updatedList, tail) } reverse(Nil, listOfElements) }
}
When we run the preceding application, we can observe the following output:
Original List = List(1, 2, 3, 4, 5, 6, 7, 8, 9) After Tail Recursion of List = List(9, 8, 7, 6, 5, 4, 3, 2, 1)