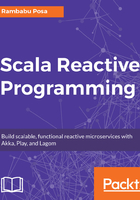
上QQ阅读APP看书,第一时间看更新
Benefits of Type classes
Scala Type classes provide us with the following benefits:
- It avoids lots of boilerplate code
- It avoids code redundancy
- It supports the DRY design principle very well
- We can write very flexible and more reusable code
- We can write extensible code
- We can write generic code easily
- It supports separation of concerns (Loosely Coupling) very well
- It encourages composition over inheritance
- It supports more type-safety
The following diagram shows the four main benefits of Scala Type classes:

Apart from Scala-based applications, Scala, Akka Toolkit, and Play Framework, source code uses Type classes extensively. For instance, the Cats library uses Type classes extensively. Please refer to this repository: https://github.com/typelevel/cats.
For instance, a Type class, Json[T] has the following operations:
trait Json[T] { def toJson(t: T) : Json def fromJson(json: Json): T }
It is a Type class which defines a contract of two functions. We can use this contract and define any number of types by using type parameters as shown here:
object JSON { implicit object StringJson extends Json[Double] { def toJson(t: String) : Json def fromJson(json: Json): String } implicit object IntJson extends Json[Int] { def toJson(t: Int) : Json def fromJson(json: Json): Int } implicit object BooleanJson extends Json[Boolean] { def toJson(t: Boolean) : Json def fromJson(json: Json): Boolean } }