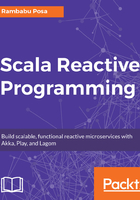
Implicit conversions
Implicit conversions are used to convert an element from a type A to another type B, when the element is used in a place where B is expected. Let's check it with the following example:
scala> val num = 10 num: Int = 10 scala> val numInString = "20" numInString: String = 20 scala> val result = num * numInString <console>:14: error: overloaded method value * with alternatives: (x: Double)Double <and> (x: Float)Float <and> (x: Long)Long <and> (x: Int)Int <and> (x: Char)Int <and> (x: Short)Int <and> (x: Byte)Int cannot be applied to (String) val result = num * numInString ^ scala> implicit def str2Int(str:String) = str.toInt str2Int: (str: String)Int scala> val result = num * numInString result: Int = 200
Explore our previously defined add() function, using Scala REPL here:
scala> def add (a: Int, b: Int) = a + b add: (a: Int, b: Int)Int scala> add(100,10) res1: Int = 110 scala> add(100,10) res2: Int = 110 scala> add(100,20) res3: Int = 120
If you observe the preceding code results, you will see that we are getting the same result for the same inputs. If we change the inputs, then we will get a different result.
Here, as the add function is dependent on its inputs only, we can say that this add function is a pure function.
There is an easy-to-understand and easy-to-remember rule of thumb about functions.
If a function does not return a result, that function has some side effects. It's not recommended you write this kind of function unless there is a specific requirement in our project:
scala> def display() = println("Hello Scala World!") display: ()Unit scala> display Hello Scala World! scala> display() Hello Scala World!
Here, the display() function does not return anything, so the Scala compiler will infer its result type as Unit. If a function does NOT take parameters, then we can make a call to it, either using parenthesis or not. Both work fine.
However, if we define our display() function as shown in the following example, then we should make a call to it without parenthesis only:
scala> def display = println("Hello Scala World!") display: Unit scala> display Hello Scala World! scala> display() <console>:12: error: Unit does not take parameters display() ^
If we define a function without parenthesis, then we should make a call to it without using parenthesis only.