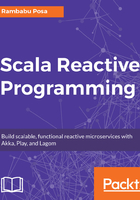
上QQ阅读APP看书,第一时间看更新
Functions are first-class citizens
In Scala, functions are first-class citizens because we can do the following things with them:
- We can use functions as values or like normal variables; we can replace a variable or value with a function
- We can assign a function literal to a variable
- We can pass one or more functions as another function's parameters
- We can return a function from another function
We will discuss the last two points in the Scala Higher-Order Functions section. Let's discuss the first two points here.
We can use a function like a normal value or variable, as shown here:
scala> def doubleIt(x: Int) = x * x doubleIt: (x: Int)Int scala> def addOne(x: Int) = x + 1 addOne: (x: Int)Int scala> val result = 10 + doubleIt(20) + addOne(49) result: Int = 460
We can assign an anonymous function to a variable, as shown in the following code snippet and use that variable where we have that function literal requirement:
val addFunction = (a: Int, b: Int) => a + b
Let us experiment this with Scala REPL:
scala> val addFunction = (a: Int, b: Int) => a + b addFunction: (Int, Int) => Int = <function2> scala> addFunction(10,20) res10: Int = 30 scala> def display(x: Int) = println("x value = " + x) display: (x: Int)Unit scala> display(addFunction(10,20)) x value = 30
As shown in the preceding example, we can use a functional literal or anonymous function as a function parameter.