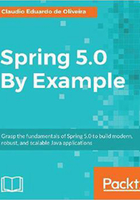
Running the application
Before we run the application, let's have a walk through our project structure.
Open the project on IntelliJ IDEA using the Import Project or Open options (both are similar), the following page will be displayed:

Then we can open or import the pom.xml file.
The following project structure should be displayed:

Open the pom.xml, we have three dependencies, spring-boot-starter-thymeleaf, spring-boot-starter-web, spring-boot-starter-test, and an interesting plugin, spring-boot-maven-plugin.
These starter dependencies are a shortcut for developers because they provide full dependencies for the module. For instance, on the spring-boot-starter-web, there is web-mvc, jackson-databind, hibernate-validator-web, and some others; these dependencies must be on the classpath to run the web applications, and starters make this task considerably easier.
Let's analyze our pom.xml, the file should look like this:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>spring-five</groupId>
<artifactId>cms</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>cms</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.8.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.16</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.7.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Also, we have a spring-boot-maven-plugin, this awesome plugin provides Spring Boot support for Maven. It enables you to package the application in a Fat-JAR, and the plugin supports the run, start, and stop goals, as well interacting with our applications.
For now, that is enough on Maven configurations; let's take a look at the Java files.
The Spring Initializr created one class for us, in general, the name of this class is artifact name plus Application, in our case CmsApplication, this class should look like this:
package springfive.cms;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class CmsApplication {
public static void main(String[] args) {
SpringApplication.run(CmsApplication.class, args);
}
}