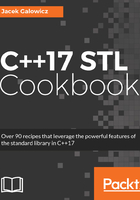
上QQ阅读APP看书,第一时间看更新
How it works...
Let’s define an example class where automatic template type deduction would be of value:
template <typename T1, typename T2, typename T3>
class my_wrapper {
T1 t1;
T2 t2;
T3 t3;
public:
explicit my_wrapper(T1 t1_, T2 t2_, T3 t3_)
: t1{t1_}, t2{t2_}, t3{t3_}
{}
/* … */
};
Okay, this is just another template class. We previously had to write the following in order to instantiate it:
my_wrapper<int, double, const char *> wrapper {123, 1.23, "abc"};
We can now just omit the template specialization part:
my_wrapper wrapper {123, 1.23, "abc"};
Before C++17, this was only possible by implementing a make function helper:
my_wrapper<T1, T2, T3> make_wrapper(T1 t1, T2 t2, T3 t3)
{
return {t1, t2, t3};
}
Using such helpers, it was possible to have a similar effect:
auto wrapper (make_wrapper(123, 1.23, "abc"));
The STL already comes with a lot of helper functions such as that one: std::make_shared, std::make_unique, std::make_tuple, and so on. In C++17, these can now mostly be regarded as obsolete. Of course, they will be provided further for compatibility reasons.