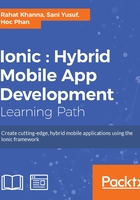
The anatomy of Ionic Project
Ionic Project is referred to in the project setup using the Ionic CLI tools locally. The later section will describe the main components of Ionic App that are relevant for the projects started using CDN files or Ionic Creator. In order to understand Ionic Project, we need to understand the project structure and the sections in the Ionic App code.
Project folder structure and important files
The Ionic App project folder structure includes folders for Cordova files, native platform code, and web content code. It also contains configuration files for dependency managers used in an Ionic App as shown in the following screenshot:

We will discuss briefly the important folders and files along with their purpose. These important folders and files are:
bower.json
(file): This is used as a configuration file for Bower, dependency managers for frontend libraries, and frameworks. The library directory for storage is written in a separate file.bowerrc
and is set towww/lib
by default.config.xml
(file): This file contains the configuration settings for the Cordova project. It is used to define meta information about the app and permissions required for the apps to be installed on specific platforms. It also includes the Cordova plugins used in the app. To read more about the different sections and tags available to be put inconfig.xml
see https://cordova.apache.org/docs/en/4.0.0/config_ref_index.md.html.gulpfile.js
(file): This is used as config script for Gulp, the build management system used for Ionic web content. It has multiple plugins used for different tasks such as concatenation, auto-installing Bower, and compiling Sass files to CSS files.ionic.project
(file): This file is used to store meta information regarding Ionic Project and associated Ionic.io cloud account services associated with it.package.json
(file): Most of the systems such as Gulp and Bower are installed and managed using Node's package manage NPM, andpackage.json
is the file to store versions of the NPM packages installed in Ionic Project.hooks
(folder): This folder contains code for Cordova hooks, which is a way to execute some code during a life cycle event in a Cordova build life cycle. Ionic utilizes theafter_prepare
hook to inject platform-specific CSS and HTML code.platforms
(folder): This folder consists of the platform subfolders that are added to Ionic Project. The subfolders contain native projects for the Ionic App.plugins
(folder): Theplugins
folder contains Cordova plugin subfolders, which include the JS library and native codebase for the specific plugins. The plugins are added and managed using the CLI commands, which we will learn about in future chapters. Cordova plugins can be developed by any developer and can be integrated using agit url/repo
.resources
(folder): A Mobile App requires static resources such as icons and splash screens. Each platform has specialized requirements regarding the sizes and format for icons. Ionic CLI provides special commands to automatically generate platform-specific resources from generic ones.scss
(folder):scss
consists of.scss
files based on the SASS framework. SASS is an extension of CSS3, which empowers the developer with multiple constructs to organize CSS code of the Ionic App. It includes features such as nested rules, variables, mixins, and more. The default fileionic.app.scss
contains important base variables such as theme colors and font paths. SASS is not necessary for developing Ionic Apps but if you know the technology, you can add yourscss
files to this folder and Gulp will automatically compile them into CSS.www
(folder): This is the most important folder that contains the actual code for Ionic App. It contains the HTML, CSS, JavaScript, and other static files such as images, fonts, and so on. Ionic Project, by default, creates subfolders under this folder forcss
,img
,js
, andtemplates
(HTML) code.
It is recommended to organize your code into different folders, one for each feature, and include all other necessary files such as controllers, directives, and services in the same folder.
Main components
The main code lies inside the www
folder. The Ionic App at its base is an AngularJS app with an Ionic module injected and multiple directives being used. We will discuss the main constructs and components in a basic Ionic App, which are the most essential.
The most important file and the starting point of your Ionic App is index.html
. It should contain important meta tags for the viewport to be able to resize properly on mobile devices. If you have created an Ionic Project using CLI, then you do not need to worry about it. If you are trying to create an Ionic App manually, please include this meta tag in the head section of your index.html
:
<meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no, width=device-width">
Also, please check the references to the Ionic bundle script or AngularJS script if the bundled library is not used. Also, please check that cordova.js
is injected as this reference will be required only after Cordova builds the app, and will give a 404 error during the development phase and testing on browsers:
<!-- ionic/angularjs js --> <script src="lib/ionic/js/ionic.bundle.js"></script> <!-- cordova script (this will be a 404 during development) --> <script src="cordova.js"></script>
The next important thing is the ng-app
directive and the value given to the attribute. The ng-app
directive should be present as an attribute on either body tag or the root HTML tag. This is required to bootstrap the Angular app using the same name in app.js
while defining the root angular module. The body tag can include any Ionic components. We will discuss some common important Ionic directives that will form the base for an Ionic App.
App.js and the root module
The next important file is the app.js
, which will be used to bootstrap the Ionic/Angular app. The name of the module should match the value given to the ng-app
attribute in index.html
.
It is also required to inject the ionic
module dependency into our root module so that we can use Ionic library directives and other constructs. We can inject any other modules into the root module, for example, the services, controllers, or custom directives that we write for our app.
In a Hybrid Mobile App, we have to call native functionalities using the Cordova plugin. It is compulsory to listen to Cordova's device ready
event, which lets us know that the native Cordova library has been loaded and our HTML code can call native functionality. Similarly, in Ionic, there is a facility to register a callback in the $ionicPlatform.ready
event, which fires after the Cordova's device ready
event. We should check the status of native plugins or call any native functionality inside this ready
event callback. The default code in the run
block of the root module when you create an Ionic App is as follows:
angular.module('starter', ['ionic']) // starter.services etc can be added .run(function($ionicPlatform) { $ionicPlatform.ready(function() { // Hide the accessory bar by default (remove this to show the accessory bar above the keyboard // for form inputs) if (window.cordova && window.cordova.plugins && window.cordova.plugins.Keyboard) { cordova.plugins.Keyboard.hideKeyboardAccessoryBar(true); cordova.plugins.Keyboard.disableScroll(true); } if (window.StatusBar) { // org.apache.cordova.statusbar required StatusBar.styleLightContent(); } }); })
Inside the ready
function we are detecting whether the keyboard plugin is present in our Ionic App, and we are hiding the keyboard accessory bar and disabling the scroll. You can change these values as per your requirements in your app.
In a basic Ionic App, the two important directives used to put content in are <ion-content>
and <ion-pane>
. Generally, the content is put in a navigation view, but navigation directives will be discussed in the next chapter. In order to create very basic apps, direct content directives can be used in the main file or by using templates for different routes.
The ion-content
directive is used to wrap the content of a view, which can also be configured to use Ionic's custom scroll view, or the built-in scrolling of the web view. It is important to note that this directive gets its own child scope and thus any models inside this directive should be referenced accordingly. Some important attributes that can be used along with this directive are given in the following table:
